一 函数式接口
@FunctionalInterface
只有一个抽象方法
有且只有一个抽象方法的接口,接口中可以包含其他的方法,包括默认方法,静态方法。如果接口中只有一个抽象方法,那么@FunctionalInterface也可以省略。
函数式接口的使用:可以作为方法的参数和返回值类型
/**
* 函数式接口
* @FunctionalInterface
* 作用:可以检测接口是否是一个函数式接口
* 是:编译成功
* 否:编译失败(接口中没有抽象方法,抽象方法的个数大于1)
*/
@FunctionalInterface
public interface MyInterface {
//定义一个抽象方法
public abstract void say();
}
//一个实现类
public class MyInterfaceImpl implements MyInterface {
@Override
public void say() {
System.out.println("say...");
}
}
//测试类
public class Test1 {
public static void show(MyInterface myInterface){
myInterface.say();
}
public static void main(String[] args) {
//调用show方法,传入MyInterfaceImpl实例
MyInterface myInterface = new MyInterfaceImpl();
show(myInterface);
//调用show方法,方法的参数是一个接口,所以可以传递接口的匿名内部类
show(new MyInterface() {
@Override
public void say() {
System.out.println("say...");
}
});
}
}
上面测试类中第一次调用show方法时候,我们是先定义了一个MyInterface接口的实现类 MyInterfaceImpl,然后创建了一个 MyInterfaceImpl的实例,作为参数传入show方法;第二次调用show方法时候,我们传入一个匿名内部类,下面我们来讲一下第三种方法
public static void main(String[] args) {
//通过接口创建一个匿名内部类,并把这个类的实例赋值个一个变量
MyInterface myInterface1=new MyInterface() {
@Override
public void say() {
System.out.println("say...");
}
};
//通过变量调用接口中的say方法
myInterface1.say();
}
1.匿名内部类
public interface Person {
public void talk();
}
public class Demo {
public static void main(String[] args) {
Person p = new Person() {
public void talk() {
System.out.println("说些什么......");
}
};
p.talk();
}
}
2.普通接口
可以有默认方法 静态方法
注意:在jdk8以前,接口中只能有抽象方法,但是到了jdk8,接口中除了抽象方法,还可以有多个默认方法。
public interface InterfaceDemo {
public abstract void run();//public 和 abstract 也可以省略
//默认方法,使用default关键字修饰
public default void sing(){
System.out.println("sing .......");
}
//默认方法,使用default关键字修饰
public default void talk(){
System.out.println("talk .......");
}
//静态方法,可以直接通过接口名来调用
public static void swim(){
System.out.println("swim....");
}
}
public class InterfaceDemoImpl implements InterfaceDemo {
@Override
public void run() {
System.out.println("run.....");
}
//注意:sing在接口中是个默认方法,用default关键字修饰,
//我们在实现类中可以对这个方法进行Override,和可以不进行Override
@Override
public void sing() {
System.out.println("Override sing.....");
}
//注意:talk在接口中是个默认方法,用default关键字修饰,
//我们在实现类中可以对这个方法进行Override,和可以不进行Override
@Override
public void talk() {
System.out.println("Override talk.....");
}
}
public class Test1 {
public static void main(String[] args) {
InterfaceDemo interfaceDemo=new InterfaceDemoImpl();
interfaceDemo.run();//输出run.....
interfaceDemo.sing();//如果在实现类中overwrite了sing方法,这里就会输出Override sing.....否则就会输出sing.....
interfaceDemo.talk();//如果在实现类中overwrite了talk方法,这里就会输出Override talk.....否则就会输出talk.....
//直接调用静态方法
InterfaceDemo.swim();//输出swim....
}
}
3.常见函数式接口
- Consumer 消费接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中对传入的参数进行消费。
- Function 计算转换接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中对传入的参数计算或转换,把结果返回
- Predicate 判断接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中对传入的参数条件判断,返回判断结果
- Supplier 生产型接口 根据其中抽象方法的参数列表和返回值类型知道,我们可以在方法中创建对象,把创建好的对象返回
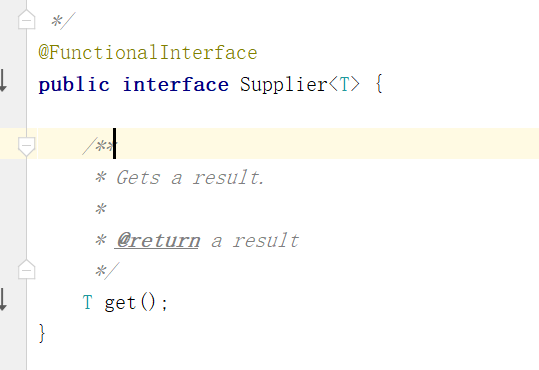
4.常用的默认方法
- and 我们在使用Predicate接口时候可能需要进行判断条件的拼接。而and方法相当于是使用&&来拼接两个判断条件 例如: 打印作家中年龄大于17并且姓名的长度大于1的作家。
List<Author> authors = getAuthors();
Stream<Author> authorStream = authors.stream();
authorStream.filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge()>17;
}
}.and(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getName().length()>1;
}
})).forEach(author -> System.out.println(author));
- or 我们在使用Predicate接口时候可能需要进行判断条件的拼接。而or方法相当于是使用||来拼接两个判断条件。 例如: 打印作家中年龄大于17或者姓名的长度小于2的作家。
// 打印作家中年龄大于17或者姓名的长度小于2的作家。
List<Author> authors = getAuthors();
authors.stream()
.filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge()>17;
}
}.or(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getName().length()<2;
}
})).forEach(author -> System.out.println(author.getName()));
- negate Predicate接口中的方法。negate方法相当于是在判断添加前面加了个! 表示取反 例如: 打印作家中年龄不大于17的作家。
// 打印作家中年龄不大于17的作家。
List<Author> authors = getAuthors();
authors.stream()
.filter(new Predicate<Author>() {
@Override
public boolean test(Author author) {
return author.getAge()>17;
}
}.negate()).forEach(author -> System.out.println(author.getAge()));
二 Lambda表达式
1.Lambda表达式引入
Lambda是JDK8中一个语法糖。他可以对某些匿名内部类的写法进行简化。它是函数式编程思想的一个重要体现。让我们不用关注是什么对象。而是更关注我们对数据进行了什么操作。
简化为如下代码
public static void main(String[] args) {
//这里把匿名内部类的名称,new关键字,以及内部的方法say签名部分都省略了
MyInterface myInterface2=()->{
System.out.println("say...");
};
myInterface2.say();
}
当实现方法内部是有一条执行语句的时候,在方法体外部的一对大括号也可以省略
public static void main(String[] args) {
//方法体只有一条执行语句,所以大括号也省略了
MyInterface myInterface3=()-> System.out.println("say...");
myInterface3.say();
}
上面的代码中,我们通过对内部类一步步简化,最终得到了lambda表达式。这是因为我们的MyInterface是一个函数式的接口,只有一个抽象方法,其实lambda表达式的实现部分就是对我们函数式接口中唯一的一个抽象方法的实现,所以,在lambda表达式中,我们不在需要方法名部分,只需要给出实现部分即可。
2. Lambda表达式语法
lambda表达式有三个主要部分构成
- 以小括号()来关闭形参,小括号中可以有放入形参列表,以逗号分开,如果没有形参,小括号中就保持空白
- 小括号()后面紧跟着箭头标记:->
- 箭头标记后是方法的实现部分,可以是一条执行语句,也可以是多条语句组成的代码块;如果是多条语句,需要放在{}中;如果是一条语句,那么{}可以省略

三 方法引用
wrappers.like(HqhBookDO::getIsbn, hqhBook.getIsbn());
// 其实就是告诉他是哪一列
like(R column, Object val)
//R为泛型,在普通wrapper中是String,在LambdaWrapper中是函数(例:Entity::getId,Entity为实体类,getId为字段id的getMethod)
类型 | 语法 | 对应Lambda表达式 |
---|---|---|
静态方法引用 | 类名::staticMethod | (args) -> 类名.staticMethod(args) |
实例方法引用 | inst::instMethod | (args) -> inst.instMethod(args) |
对象方法引用 | 类名::instMethod | (inst,args) -> inst.instMethod(args) |
构建方法引用 | 类名::new | (args) -> new 类名(args) |
四 stream流
@Data
@NoArgsConstructor
@AllArgsConstructor
@EqualsAndHashCode//用于后期的去重使用
public class Author {
//id
private Long id;
//姓名
private String name;
//年龄
private Integer age;
//简介
private String intro;
//作品
private List<Book> books;
}
private static List<Author> getAuthors() {
//数据初始化
Author author = new Author(1L,"蒙多",33,"一个从菜刀中明悟哲理的祖安人",null);
Author author2 = new Author(2L,"亚拉索",15,"狂风也追逐不上他的思考速度",null);
Author author3 = new Author(3L,"易",14,"是这个世界在限制他的思维",null);
Author author4 = new Author(3L,"易",14,"是这个世界在限制他的思维",null);
//书籍列表
List<Book> books1 = new ArrayList<>();
List<Book> books2 = new ArrayList<>();
List<Book> books3 = new ArrayList<>();
books1.add(new Book(1L,"刀的两侧是光明与黑暗","哲学,爱情",88,"用一把刀划分了爱恨"));
books1.add(new Book(2L,"一个人不能死在同一把刀下","个人成长,爱情",99,"讲述如何从失败中明悟真理"));
books2.add(new Book(3L,"那风吹不到的地方","哲学",85,"带你用思维去领略世界的尽头"));
books2.add(new Book(3L,"那风吹不到的地方","哲学",85,"带你用思维去领略世界的尽头"));
books2.add(new Book(4L,"吹或不吹","爱情,个人传记",56,"一个哲学家的恋爱观注定很难把他所在的时代理解"));
books3.add(new Book(5L,"你的剑就是我的剑","爱情",56,"无法想象一个武者能对他的伴侣这么的宽容"));
books3.add(new Book(6L,"风与剑","个人传记",100,"两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?"));
books3.add(new Book(6L,"风与剑","个人传记",100,"两个哲学家灵魂和肉体的碰撞会激起怎么样的火花呢?"));
author.setBooks(books1);
author2.setBooks(books2);
author3.setBooks(books3);
author4.setBooks(books3);
List<Author> authorList = new ArrayList<>(Arrays.asList(author,author2,author3,author4));
return authorList;
}
1.创建流
单列集合: 集合对象.stream()
List<Author> authors = getAuthors();
Stream<Author> stream = authors.stream();
数组:Arrays.stream(数组)
或者使用Stream.of
来创建
Integer[] arr = {1,2,3,4,5};
Stream<Integer> stream = Arrays.stream(arr);
Stream<Integer> stream2 = Stream.of(arr);
双列集合:转换成单列集合后再创建
Map<String,Integer> map = new HashMap<>();
map.put("蜡笔小新",19);
map.put("黑子",17);
map.put("日向翔阳",16);
Stream<Map.Entry<String, Integer>> stream = map.entrySet().stream();
2.中间操作
filter 过滤
可以对流中的元素进行条件过滤,符合过滤条件的才能继续留在流中。
例如:
打印所有姓名长度大于1的作家的姓名
List<Author> authors = getAuthors();
authors.stream()
.filter(author -> author.getName().length()>1)
.forEach(author -> System.out.println(author.getName()));
map 计算或转换
可以把对流中的元素进行计算或转换。
例如:
打印所有作家的姓名
List<Author> authors = getAuthors();
authors
.stream()
.map(author -> author.getName())
.forEach(name->System.out.println(name));
// 打印所有作家的姓名
List<Author> authors = getAuthors();
// authors.stream()
// .map(author -> author.getName())
// .forEach(s -> System.out.println(s));
authors.stream()
.map(author -> author.getAge())
.map(age->age+10)
.forEach(age-> System.out.println(age));
distinct 去重
可以去除流中的重复元素。
例如:
打印所有作家的姓名,并且要求其中不能有重复元素。
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.forEach(author -> System.out.println(author.getName()));
注意:distinct方法是依赖Object的equals方法来判断是否是相同对象的。所以需要注意重写equals方法。
sorted 排序
可以对流中的元素进行排序。
例如:
对流中的元素按照年龄进行降序排序,并且要求不能有重复的元素。
List<Author> authors = getAuthors();
// 对流中的元素按照年龄进行降序排序,并且要求不能有重复的元素。
authors.stream()
.distinct()
.sorted()
.forEach(author -> System.out.println(author.getAge()));
List<Author> authors = getAuthors();
// 对流中的元素按照年龄进行降序排序,并且要求不能有重复的元素。
authors.stream()
.distinct()
.sorted((o1, o2) -> o2.getAge()-o1.getAge())
.forEach(author -> System.out.println(author.getAge()));
注意:如果调用空参的sorted()方法,需要流中的元素是实现了Comparable。
limit 限制
可以设置流的最大长度,超出的部分将被抛弃。
例如:
对流中的元素按照年龄进行降序排序,并且要求不能有重复的元素,然后打印其中年龄最大的两个作家的姓名。
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted()
.limit(2)
.forEach(author -> System.out.println(author.getName()));
skip 跳过
跳过流中的前n个元素,返回剩下的元素
例如:
打印除了年龄最大的作家外的其他作家,要求不能有重复元素,并且按照年龄降序排序。
// 打印除了年龄最大的作家外的其他作家,要求不能有重复元素,并且按照年龄降序排序。
List<Author> authors = getAuthors();
authors.stream()
.distinct()
.sorted()
.skip(1)
.forEach(author -> System.out.println(author.getName()));
flatMap 对象转换 .stream()
map只能把一个对象转换成另一个对象来作为流中的元素。而flatMap可以把一个对象转换成多个对象作为流中的元素。
例一:
打印所有书籍的名字。要求对重复的元素进行去重。
// 打印所有书籍的名字。要求对重复的元素进行去重。
List<Author> authors = getAuthors();
authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.forEach(book -> System.out.println(book.getName()));
例二:
打印现有数据的所有分类。要求对分类进行去重。不能出现这种格式:哲学,爱情
// 打印现有数据的所有分类。要求对分类进行去重。不能出现这种格式:哲学,爱情 爱情
List<Author> authors = getAuthors();
authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.flatMap(book -> Arrays.stream(book.getCategory().split(",")))
.distinct()
.forEach(category-> System.out.println(category));
终结操作
forEach 变量
对流中的元素进行遍历操作,我们通过传入的参数去指定对遍历到的元素进行什么具体操作。
例子:
输出所有作家的名字
// 输出所有作家的名字
List<Author> authors = getAuthors();
authors.stream()
.map(author -> author.getName())
.distinct()
.forEach(name-> System.out.println(name));
count 统计数量
可以用来获取当前流中元素的个数。
例子:
打印这些作家的所出书籍的数目,注意删除重复元素。
// 打印这些作家的所出书籍的数目,注意删除重复元素。
List<Author> authors = getAuthors();
long count = authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.count();
System.out.println(count);
max&min 最值
可以用来或者流中的最值。
例子:
分别获取这些作家的所出书籍的最高分和最低分并打印。
// 分别获取这些作家的所出书籍的最高分和最低分并打印。
//Stream<Author> -> Stream<Book> ->Stream<Integer> ->求值
List<Author> authors = getAuthors();
Optional<Integer> max = authors.stream()
.flatMap(author -> author.getBooks().stream())
.map(book -> book.getScore())
.max((score1, score2) -> score1 - score2);
Optional<Integer> min = authors.stream()
.flatMap(author -> author.getBooks().stream())
.map(book -> book.getScore())
.min((score1, score2) -> score1 - score2);
System.out.println(max.get());
System.out.println(min.get());
collect 转换集合
把当前流转换成一个集合。
例子:
获取一个存放所有作者名字的List集合。
// 获取一个存放所有作者名字的List集合。
List<Author> authors = getAuthors();
List<String> nameList = authors.stream()
.map(author -> author.getName())
.collect(Collectors.toList());
System.out.println(nameList);
获取一个所有书名的Set集合。
// 获取一个所有书名的Set集合。
List<Author> authors = getAuthors();
Set<Book> books = authors.stream()
.flatMap(author -> author.getBooks().stream())
.collect(Collectors.toSet());
System.out.println(books);
获取一个Map集合,map的key为作者名,value为List
// 获取一个Map集合,map的key为作者名,value为List<Book>
List<Author> authors = getAuthors();
Map<String, List<Book>> map = authors.stream()
.distinct()
.collect(Collectors.toMap(author -> author.getName(), author -> author.getBooks()));
System.out.println(map);
查找与匹配
anyMatch
可以用来判断是否有任意符合匹配条件的元素,结果为boolean类型。
例子:
判断是否有年龄在29以上的作家
// 判断是否有年龄在29以上的作家
List<Author> authors = getAuthors();
boolean flag = authors.stream()
.anyMatch(author -> author.getAge() > 29);
System.out.println(flag);
allMatch
可以用来判断是否都符合匹配条件,结果为boolean类型。如果都符合结果为true,否则结果为false。
例子:
判断是否所有的作家都是成年人
// 判断是否所有的作家都是成年人
List<Author> authors = getAuthors();
boolean flag = authors.stream()
.allMatch(author -> author.getAge() >= 18);
System.out.println(flag);
noneMatch
可以判断流中的元素是否都不符合匹配条件。如果都不符合结果为true,否则结果为false
例子:
判断作家是否都没有超过100岁的。
// 判断作家是否都没有超过100岁的。
List<Author> authors = getAuthors();
boolean b = authors.stream()
.noneMatch(author -> author.getAge() > 100);
System.out.println(b);
findAny
获取流中的任意一个元素。该方法没有办法保证获取的一定是流中的第一个元素。
例子:
获取任意一个年龄大于18的作家,如果存在就输出他的名字
// 获取任意一个年龄大于18的作家,如果存在就输出他的名字
List<Author> authors = getAuthors();
Optional<Author> optionalAuthor = authors.stream()
.filter(author -> author.getAge()>18)
.findAny();
optionalAuthor.ifPresent(author -> System.out.println(author.getName()));
findFirst
获取流中的第一个元素。
例子:
获取一个年龄最小的作家,并输出他的姓名。
// 获取一个年龄最小的作家,并输出他的姓名。
List<Author> authors = getAuthors();
Optional<Author> first = authors.stream()
.sorted((o1, o2) -> o1.getAge() - o2.getAge())
.findFirst();
first.ifPresent(author -> System.out.println(author.getName()));
reduce归并 就算
对流中的数据按照你指定的计算方式计算出一个结果。(缩减操作)
reduce的作用是把stream中的元素给组合起来,我们可以传入一个初始值,它会按照我们的计算方式依次拿流中的元素和初始化值进行计算,计算结果再和后面的元素计算。
reduce两个参数的重载形式内部的计算方式如下:
T result = identity;
for (T element : this stream)
result = accumulator.apply(result, element)
return result;
其中identity就是我们可以通过方法参数传入的初始值,accumulator的apply具体进行什么计算也是我们通过方法参数来确定的。
例子:
使用reduce求所有作者年龄的和
// 使用reduce求所有作者年龄的和
List<Author> authors = getAuthors();
Integer sum = authors.stream()
.distinct()
.map(author -> author.getAge())
.reduce(0, (result, element) -> result + element);
System.out.println(sum);
使用reduce求所有作者中年龄的最大值
// 使用reduce求所有作者中年龄的最大值
List<Author> authors = getAuthors();
Integer max = authors.stream()
.map(author -> author.getAge())
.reduce(Integer.MIN_VALUE, (result, element) -> result < element ? element : result);
System.out.println(max);
使用reduce求所有作者中年龄的最小值
// 使用reduce求所有作者中年龄的最小值
List<Author> authors = getAuthors();
Integer min = authors.stream()
.map(author -> author.getAge())
.reduce(Integer.MAX_VALUE, (result, element) -> result > element ? element : result);
System.out.println(min);
reduce一个参数的重载形式内部的计算
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
如果用一个参数的重载方法去求最小值代码如下:
// 使用reduce求所有作者中年龄的最小值
List<Author> authors = getAuthors();
Optional<Integer> minOptional = authors.stream()
.map(author -> author.getAge())
.reduce((result, element) -> result > element ? element : result);
minOptional.ifPresent(age-> System.out.println(age));
五 并行流
当流中有大量元素时,我们可以使用并行流去提高操作的效率。其实并行流就是把任务分配给多个线程去完全。如果我们自己去用代码实现的话其实会非常的复杂,并且要求你对并发编程有足够的理解和认识。而如果我们使用Stream的话,我们只需要修改一个方法的调用就可以使用并行流来帮我们实现,从而提高效率。
parallel方法可以把串行流转换成并行流。
private static void test28() {
Stream<Integer> stream = Stream.of(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
Integer sum = stream.parallel()
.peek(new Consumer<Integer>() {
@Override
public void accept(Integer num) {
System.out.println(num+Thread.currentThread().getName());
}
})
.filter(num -> num > 5)
.reduce((result, ele) -> result + ele)
.get();
System.out.println(sum);
}
也可以通过parallelStream直接获取并行流对象。
List<Author> authors = getAuthors();
authors.parallelStream()
.map(author -> author.getAge())
.map(age -> age + 10)
.filter(age->age>18)
.map(age->age+2)
.forEach(System.out::println);
注意事项
- 惰性求值(如果没有终结操作,没有中间操作是不会得到执行的)
- 流是一次性的(一旦一个流对象经过一个终结操作后。这个流就不能再被使用)
- 不会影响原数据(我们在流中可以多数据做很多处理。但是正常情况下是不会影响原来集合中的元素的。这往往也是我们期望的)
六 Optional
我们在编写代码的时候出现最多的就是空指针异常。所以在很多情况下我们需要做各种非空的判断。
例如:
Author author = getAuthor();
if(author!=null){
System.out.println(author.getName());
}
尤其是对象中的属性还是一个对象的情况下。这种判断会更多。
Author author = getAuthor();
Optional<Author> authorOptional = Optional.ofNullable(author);
Optional<Author> optionalAuthor = Optional.of(getAuthors().get(0));
Optional<Author> optionalAuthor1 =authors.stream().filter(i -> i.getId() == 1).findFirst();
1.安全获取值
如果我们期望安全的获取值。我们不推荐使用get方法,而是使用Optional提供的以下方法。
- orElseGet 获取数据并且设置数据为空时的默认值。如果数据不为空就能获取到该数据。如果为空则根据你传入的参数来创建对象作为默认值返回。
Optional<Author> authorOptional = Optional.ofNullable(getAuthor());
Author author1 = authorOptional.orElseGet(() -> new Author());
- orElseThrow 获取数据,如果数据不为空就能获取到该数据。如果为空则根据你传入的参数来创建异常抛出。
Optional<Author> authorOptional = Optional.ofNullable(getAuthor());
try {
Author author = authorOptional.orElseThrow((Supplier<Throwable>) () -> new RuntimeException("author为空"));
System.out.println(author.getName());
} catch (Throwable throwable) {
throwable.printStackTrace();
}
Comments NOTHING